Create your custom snippet in VS Code
I recently started learning React and found myself repeating the same lines of code whenever I want to create a new component. Be either a class component or a functional component. I continued repeating the lines of code for like a month but then got tired.
Finally, I decided to look out on the web on how to create my own VS Code snippets. I found helpful and straightforward results and eventually, I created my custom snippet😁.
Open Visual Studio Code and go to settings and click on User Snippets
as shown below
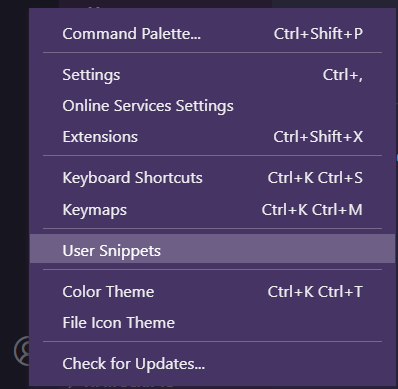
Settings > User Snippets
Then choose Add New Global Snippet
and give it any name of your choice.
We have our snippet file(JSON) created with an object. Inside the object, there is a comment which I’ll uncomment and paste here to make it more readable and understandable.
Place your global snippets here. Each snippet is defined under a snippet name and has a scope, prefix, body, and description. Add comma-separated ids of the languages where the snippet is applicable in the scope field. If the scope is left empty or omitted, the snippet gets applied to all languages. The prefix is what is used to trigger the snippet and the body will be expanded and inserted. Possible variables are: $1, $2 for tab stops, $0 for the final cursor position, and ${1:label}, ${2:another} for placeholders.
Placeholders with the same ids are connected.
Example: “Print to console”: {
“scope”: “javascript,typescript”,
“prefix”: “log”,
“body”: [
“console.log(‘$1’);”,
],
“description”: “Log output to console”
},
Looking at the example given which is a snippet for JavaScript’s console.log()
. To use this snippet, all you have to type in the code editor is just log
and press enter.
Let’s add more snippets. Like I said earlier, I recently started learning React. We’ll create two snippets: a functional component and a class component. I have emptied the JSON object. This is what I have now:
{
}
Creating the functional component snippet first, this is what I’ll like to achieve
import React from "react"
const name = (props) => {
return (
<div>
</div>
)
};
export default name;
This is the code for the snippet
{
"React Functional Component": {
"prefix": "ReactFunc",
"body": [
"import React from \"react\"",
"",
"const ${1:name} = (props) => {",
" return (",
" <div>",
" $2",
" </div>",
" )",
"};",
"",
"export default ${1:name};",
""
],
"description": "React Functional Component"
}
}
- The snippet is named React Functional Component.
- A
prefix
key with a string propertyReactFunc
- A
body
key with an array of each line of the code we want to render. - And then a
description
key describing the snippet.
Whenever we want to use the snippet, we type the prefix property which is ReactFunc
and press enter. Sometimes, just typing RF
and pressing enter works too.
Let’s add the second snippet to the JSON object, a class component.
This is what I will like to achieve
import React, { Component } from "react";
class name extends Component {
render(){
return(
<div>
</div>
)
}
}
export default name;
We add this to the JSON object
{
{
the first snippet here...
},
"React Class Component": {
"prefix": "ReactClass",
"body": [
"import React, { Component } from \"react\";",
"",
"class ${1:name} extends Component {",
" render(){",
" return(",
" <div>",
" $2",
" </div>",
" )",
" }",
"}",
"",
"export default ${1:name};"
],
"description": "React Class Component"
}
}
Whenever I want to create a React class component, I’ll have to do is type ReactClass
.
To sum it up, our snippet file will looks like this
{
"React Functional Component": {
"prefix": "ReactFunc",
"body": [
"import React from \"react\"",
"",
"const ${1:name} = (props) => {",
" return (",
" <div>",
" $2",
" </div>",
" )",
"};",
"",
"export default ${1:name};",
""
],
"description": "React Functional Component"
},
"React Class Component": {
"prefix": "ReactClass",
"body": [
"import React, { Component } from \"react\";",
"",
"class ${1:name} extends Component {",
" render(){",
" return(",
" <div>",
" $2",
" </div>",
" )",
" }",
"}",
"",
"export default ${1:name};"
],
"description": "React Class Component"
}
}
}
You can also work smarter by using an online snippet generator like snippet.generator.app. It supports VS Code, Atom, and Sublime Text.
To read more about VS code snippets, check Snippets in Visual Studio.)